npm packages
Release a new npm package version
A basic example of how to release a new npm package version with Optic.
Setting up the Optic release action
name: release
on:
workflow_dispatch:
inputs:
semver:
description: 'The semver to use'
required: true
pull_request:
types: [closed]
jobs:
release:
runs-on: ubuntu-latest
permissions:
contents: write
issues: write
pull-requests: write
steps:
- uses: nearform-actions/optic-release-automation-action@v4
with:
npm-token: ${{secrets.NPM_TOKEN}}
semver: ${{ github.event.inputs.semver }}
Setting up npm token
You can get an npm token by following this instructions
Release a new npm package version with 2FA enabled
Securely release npm packages with Optic's 2FA-enabled workflow. Optic automates the workflow, seamlessly integrating 2FA verification to enhance the security of your npm packages.
Setting up Optic mobile app
In order to set up Optic, the first thing you need to do is generate a 2FA token for your npm account, and then save it to your mobile device using the Optic app. This secret token is never transmitted to any 3rd party servers.
Instead, Optic mobile app requests the backend to generate a token that corresponds to your 2FA token and your mobile device. This Optic token is then stored on the device and used during the release process. The Optic token must also be saved in the repository secrets in order to be used by the release workflow action.
You can see more information at the mobile app docs
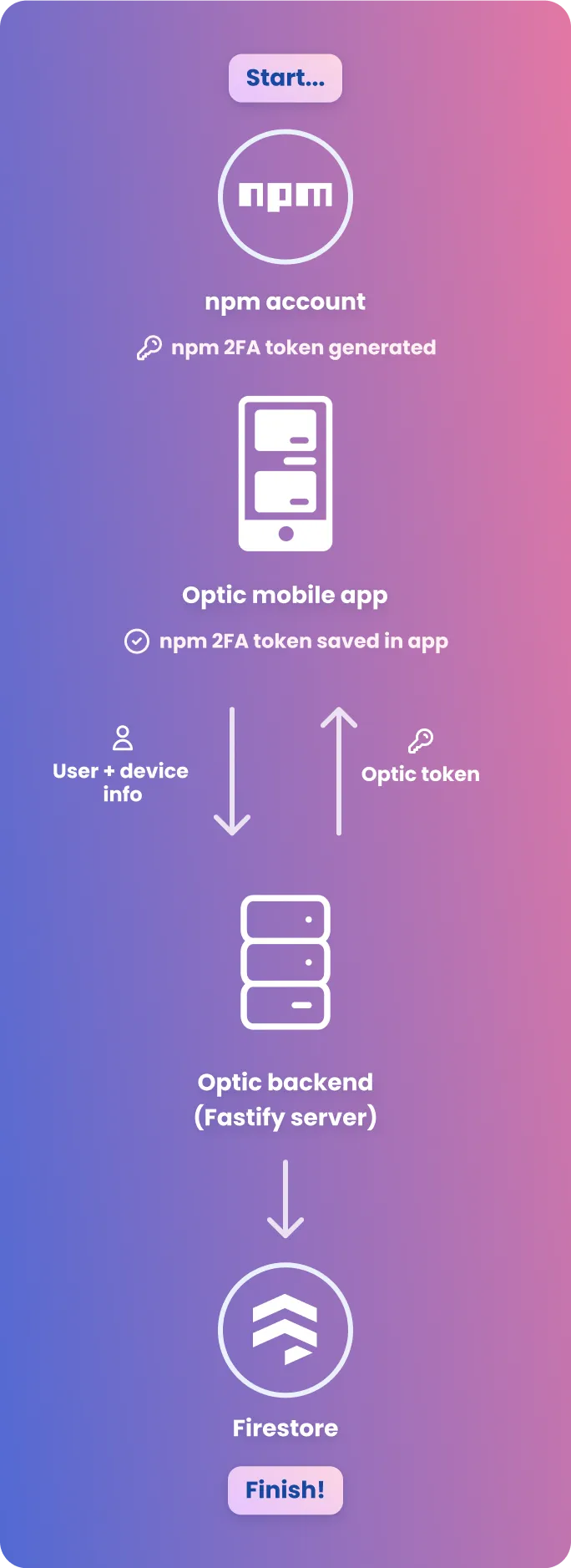
Screenshots of Optic mobile application
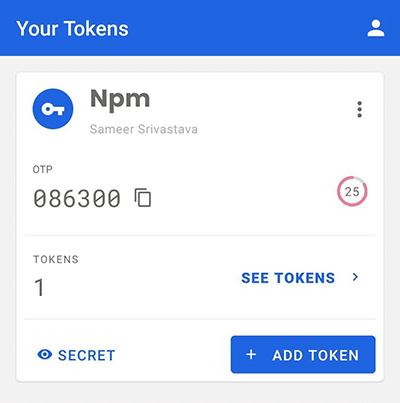
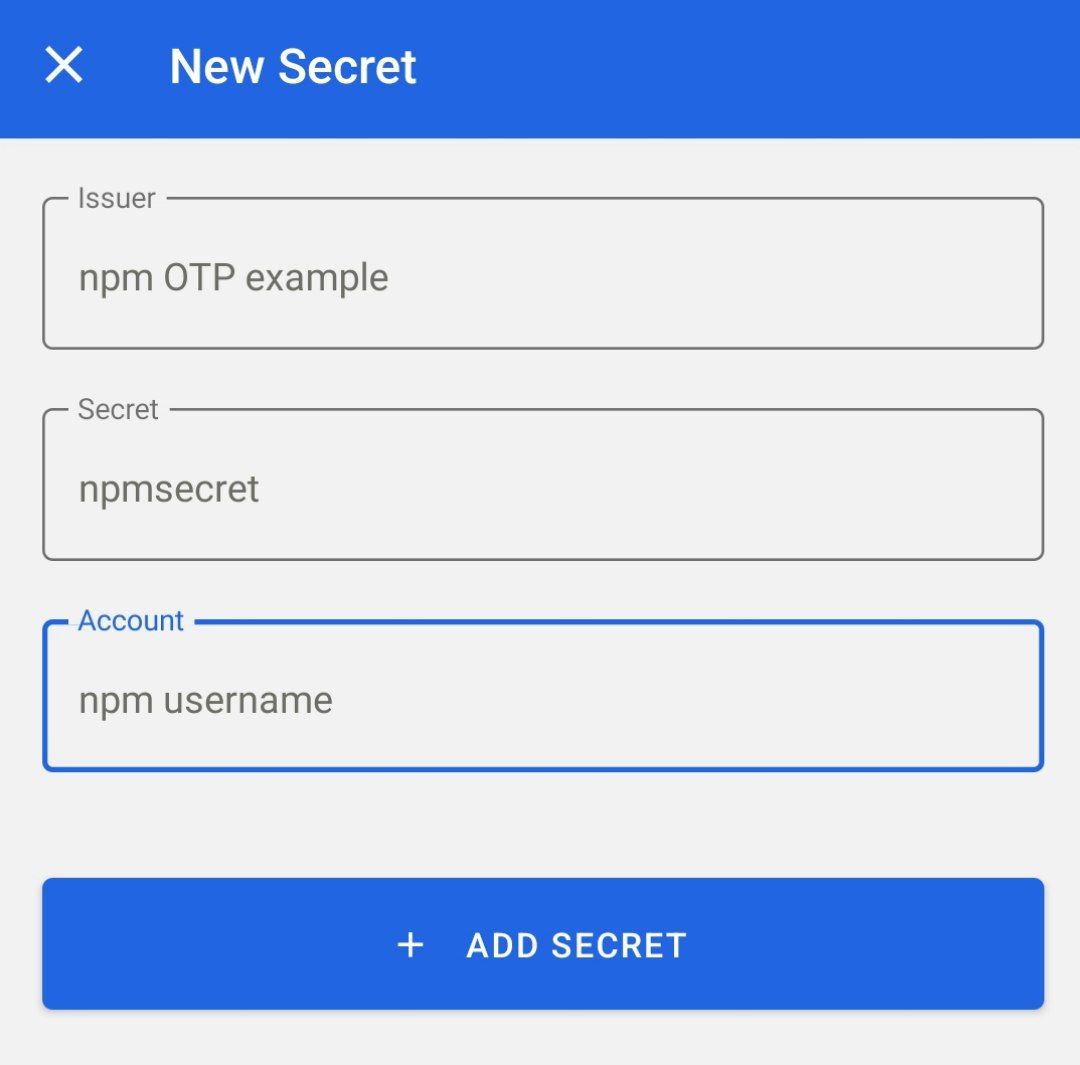
Setting up the Optic release action
name: release
on:
workflow_dispatch:
inputs:
semver:
description: 'The semver to use'
required: true
tag:
description: 'The npm tag'
required: false
default: 'latest'
pull_request:
types: [closed]
jobs:
release:
runs-on: ubuntu-latest
permissions:
contents: write
issues: write
pull-requests: write
steps:
- uses: nearform-actions/optic-release-automation-action@v4
with:
npm-token: ${{ secrets.NPM_TOKEN }}
optic-token: ${{ secrets.OPTIC_TOKEN }}
semver: ${{ github.event.inputs.semver }}
npm-tag: ${{ github.event.inputs.tag }}
Release a new npm package version with Provenance
Ensure the integrity and authenticity of your packages with npm's provenance feature, giving users confidence in the origin and security of your code. Enhance transparency and compliance with industry standards by easily enabling provenance in your release process.
name: release
on:
workflow_dispatch:
inputs:
semver:
description: 'The semver to use'
required: true
pull_request:
types: [closed]
jobs:
release:
runs-on: ubuntu-latest
permissions:
contents: write
issues: write
pull-requests: write
# add this permission which is required for provenance
id-token: write
steps:
- uses: nearform-actions/optic-release-automation-action@v4
with:
npm-token: ${{ secrets.NPM_TOKEN }}
optic-token: ${{ secrets.OPTIC_TOKEN }}
semver: ${{ github.event.inputs.semver }}
# add this to activate the action's provenance feature
provenance: true
GitHub actions
Release a new GitHub action version
This recipe automates the release process of a GitHub action.
Setting up the Optic release action
name: release
on:
workflow_dispatch:
inputs:
semver:
description: 'The semver to use'
required: true
pull_request:
types: [closed]
jobs:
release:
runs-on: ubuntu-latest
permissions:
contents: write
issues: write
pull-requests: write
steps:
- uses: nearform-actions/optic-release-automation-action@v4
with:
semver: ${{ github.event.inputs.semver }}
# If you want to keep the major and minor versions git tags
# synced to the latest appropriate commit
sync-semver-tags: true
Release a new GitHub action version with a custom build command
This recipe allows you to automate the release process of a GitHub action with a custom build command.
Setting up the Optic release action
name: release
on:
workflow_dispatch:
inputs:
semver:
description: 'The semver to use'
required: true
pull_request:
types: [closed]
jobs:
release:
runs-on: ubuntu-latest
permissions:
contents: write
issues: write
pull-requests: write
steps:
- uses: nearform-actions/optic-release-automation-action@v4
with:
semver: ${{ github.event.inputs.semver }}
sync-semver-tags: true
build-command: |
npm ci
npm run build
Other Applications
When Optic creates a new release, you might want to, for example, deploy the application to production. In this case, you can use the trigger below in your production deployment workflow:
on:
release:
types:
- published